Need some more help...
Need some more help...
Rockbomb
Dog fucker (but in a good way now)

2009 Nov 14 • 2045
|
I can't for the life of me figure out how to get this to work... I've been trying for the last week, but to no avail.
So basically the program I'm supposed to write has a textbox that users may input a number between 20-100 into. Once they press a button, it will add that number to a listbox. It will also check to see if that number has already been put into the first listbox, and if it hasn't, it will add it into a second listbox (so the first listbox will have every inputted number in it, and the second one will have every UNIQUE number in it).
The part I'm having trouble with is determining whether a number is unique or not.
Here's the code I have so far... the addUnique function is the one that isn't working:
code Public Class frmDupElim Dim x(19) As Integer Dim count As Integer = 0 Private Sub btnAdd_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnAdd.Click If txtInput.Text = Nothing Then MessageBox.Show("Please enter a number.") Else If Convert.ToInt32(txtInput.Text) > 9 And Convert.ToInt32(txtInput.Text) < 101 And count < 20 Then x(count) = Convert.ToInt32(txtInput.Text) addUnique() addEntered() txtInput.Clear() txtInput.Focus() count += 1 Else MessageBox.Show("You must enter a number between 10 and 100.") End If End If End Sub Sub addEntered() If count < 20 Then lstEntered.Items.Add(Convert.ToString(x(count))) lblCounter.Text = Convert.ToString(count + 1) Else MessageBox.Show("You can not enter more than 20 numbers.") End If End Sub Sub addUnique() Dim y As Boolean = False For counter As Integer = 0 To 19 If x(count) = x(counter) Then y = True End If Next If y = False Then lstUnique.Items.Add(Convert.ToString(x(count))) End If End Sub Private Sub btnClear_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnClear.Click lblCounter.Text = "0" count = 0 lstEntered.Items.Clear() lstUnique.Items.Clear() End Sub End Class
|
|
|
|
≡
|
2012 Mar 16 at 04:17 UTC
|
|
|
Rockbomb
Dog fucker (but in a good way now)

2009 Nov 14 • 2045
|
Oh, and I should have also mentioned that I'm supposed to do this using a single dimensional array.
|
|
|
|
≡
|
2012 Mar 16 at 04:18 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
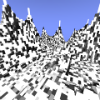

2007 Oct 19 • 5486
57,583 ₧
|
Something like for( i in array ) if array == int break; that would iterate over the array and when it finds a match, would stop, indicating that there is a match.
|
|
|
|
≡
|
2012 Mar 16 at 16:57 UTC
|
|
|
Rockbomb
Dog fucker (but in a good way now)

2009 Nov 14 • 2045
|
Down Rodeo said: Something like for( i in array ) if array == int break; that would iterate over the array and when it finds a match, would stop, indicating that there is a match.
Isn't that essentially what I already have? Except, instead of using a break, I change the boolean value of y to true. Then I check that boolean value, and if it is false, I add the number to the second listbox.
Edit: To be honest, I can't figure out why my current code doesn't work... what's wrong with it? Imo, it should work :o
|
|
|
|
≡
|
2012 Mar 17 at 21:48 UTC
— Ed. 2012 Mar 17 at 21:49 UTC
|
|
|
Rockbomb
Dog fucker (but in a good way now)

2009 Nov 14 • 2045
|
Also, I don't understand "For(i in array)".
Maybe it's just cuz vb.net is retarded, but I don't think you can set it up with that format.
The only format for a vb.net for loop is:
For value as integer = x to x
do this stuff
next
|
|
|
|
≡
|
2012 Mar 17 at 21:53 UTC
|
|
|
|
Rockbomb said: Also, I don't understand "For(i in array)".
It iterates through the array elements. So:
php code <?php $myarray = array[50,100,150,299,863]; for($i in $myarray) { print $i; } //$i = 50,100,150,299,863 ?>
That is not valid php by the way. But I hope you get the point.
|
|
|
|
≡
|
2012 Mar 17 at 23:58 UTC
— Ed. 2012 Mar 18 at 00:01 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
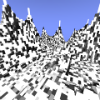

2007 Oct 19 • 5486
57,583 ₧
|
Yeah it was pseudocode. I have never used VB.NET so really I can't help you with actual syntax, only ideas. If you ever felt like using Python (which you really ought to someday, my supervisor has converted me) you can do stuff like that. So in short yes, perhaps your code does the right thing, but I am not sure.
|
|
|
|
≡
|
2012 Mar 17 at 23:58 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
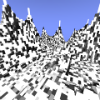

2007 Oct 19 • 5486
57,583 ₧
|
OH OH OH I got it! Right everyone, now begins the "find the bug" game. It's really cool.
|
|
|
|
≡
|
2012 Mar 18 at 00:01 UTC
|
|
|
Rockbomb
Dog fucker (but in a good way now)

2009 Nov 14 • 2045
|
Because Sprinkles made me type it out for him on Steam, I'm gonna post the exact text for this problem from my book:
Quote: Use a one-dimensional array to solve the following problem: Read in 20 numbers, each of which is between 10 and 100, inclusive. As each number is read, display it in numbersEnteredListbox and, if it's not a duplicate of a number already read, display it in uniqueValueListBox. Provide for the "Worst case" (in which all 20 numbers are different). Use the smallest possible array to solve this problem.
|
|
|
|
≡
|
2012 Mar 18 at 00:16 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
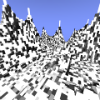

2007 Oct 19 • 5486
57,583 ₧
|
Actually, I've not found it. I originally thought that having x(counter) = x(array) was the issue but as it turns out VB uses = for equality and assignment! Retarded! So I guess I'm going to have to look again.
|
|
|
|
≡
|
2012 Mar 18 at 01:03 UTC
|
|
|
|
C# code using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace forRB { public partial class Form1 : Form { public Form1() { InitializeComponent(); } int[] numbers = new int[20]; int counter = 0; private void input_KeyUp(object sender, KeyEventArgs e) { if (e.KeyCode == Keys.Enter) { int bob; bool result = Int32.TryParse(input.Text, out bob); if (!result) { input.Text = ""; return; } else { if (Convert.ToInt32(input.Text) >= 10 && Convert.ToInt32(input.Text) <= 100) { if (counter < 20) { inclusiveLB.Items.Add(input.Text); bool repeated = false; foreach(int i in numbers) { if(i == Convert.ToInt32(input.Text)) { repeated = true; break; } } if (repeated == false) { uniqueLB.Items.Add(input.Text); } else { uniqueLB.Items.RemoveAt(uniqueLB.Items.IndexOf(input.Text)); } numbers[counter] = Convert.ToInt32(input.Text); input.Text = ""; } else { input.Text = ""; } } else { MessageBox.Show("You must enter a number between 10 and 100." + Environment.NewLine + "You entered \"" + input.Text + "\"", "Number Out of Range", MessageBoxButtons.OK); } } counter++; } else if (e.KeyCode == Keys.Escape) { inclusiveLB.Items.Clear(); uniqueLB.Items.Clear(); input.Text = ""; counter = 0; } } } }
|
|
|
|
≡
|
2012 Mar 18 at 06:01 UTC
|
|
|
Rockbomb
Dog fucker (but in a good way now)

2009 Nov 14 • 2045
|
With much thanks to Sprinkles, I've finally got a working program... what a bitch this was.
The key, for whatever reason, was to use a for each statement, rather than just a for statement. I still am unsure as to why the for statement didn't give the same results, but nonetheless, here's what I ended up with:
code Public Class frmDupElim Dim x(19) As Integer Dim count As Integer = 0 Private Sub btnAdd_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnAdd.Click If txtInput.Text = Nothing Then MessageBox.Show("Please enter a number.") Else If Convert.ToInt32(txtInput.Text) > 9 And Convert.ToInt32(txtInput.Text) < 101 And count < 20 Then addUnique() x(count) = Convert.ToInt32(txtInput.Text) addEntered() txtInput.Clear() txtInput.Focus() count += 1 Else MessageBox.Show("You must enter a number between 10 and 100.") End If End If End Sub Sub addEntered() If count < 20 Then lstEntered.Items.Add(Convert.ToString(x(count))) lblCounter.Text = Convert.ToString(count + 1) Else MessageBox.Show("You can not enter more than 20 numbers.") End If End Sub Sub addUnique() Dim y As Boolean = False For Each i As Integer In x If i = Convert.ToInt32(txtInput.Text) Then y = True Exit For End If Next If y = False Then lstUnique.Items.Add(txtInput.Text) End If End Sub Private Sub btnClear_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnClear.Click lblCounter.Text = "0" count = 0 lstEntered.Items.Clear() lstUnique.Items.Clear() Array.Clear(x, 0, 19) End Sub End Class
I also noticed that I was adding the text from the input box into the array before I was checking to see if that value was already in the array. So no matter what, it would always think that the value was in the array, even if it wasn't unique.
|
|
|
|
≡
|
2012 Mar 18 at 06:45 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
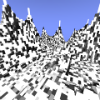

2007 Oct 19 • 5486
57,583 ₧
|
Look up For and For Each to see if there's a behavioural difference between them. VB seems like a horrid language.
|
|
|
|
≡
|
2012 Mar 18 at 17:37 UTC
|
|
|
Rockbomb
Dog fucker (but in a good way now)

2009 Nov 14 • 2045
|
Down Rodeo said: VB seems like a horrid language.
I can confirm that it is.
I just looked into what the difference between a for next and a for each would be... my findings were rather disappointing, though. Structurally, they both do the exact same thing. The only difference between them is the obvious that a for next loop is cycles through for a predetermined amount of times based on the numbers you give it, where the for each loop will start at the beginning of an array and go through every item until there are no more items.
Here's the structure for a for each:
Quote: For Each element [ As datatype ] In group
[ statements ]
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ element ]
And here's for a for next:
For counter [ As datatype ] = start To end [ Step step ]
[ statements ]
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ counter ]
|
|
|
|
≡
|
2012 Mar 18 at 17:50 UTC
|
|
|
Page [1]
|
|