Getting started in C++ - WARNING: may contain noob questions
Getting started in C++ - WARNING: may contain noob questions
|
So I decided that I'm going to start with a more complex language now so here I am taking my first steps in C++ (I wanted to go with C at first but the tutorials I checked recommended C++). I'm now following this tutorial (in case you know a better one, please share the info).
So this is now the topic where I'll make all the noob questions about it. To start things off:
In pascal I only had two functions to read an input from the user: read() and readln() (readln=read + new line so basically it's just 1 function) where you write the variable into the brackets.
But now I'm only in my first lesson and I've already got 3 different read functions: cin>> variable, cin.get() and cin.ignore() and the latter two were not really properly explained.
So it says that
cin>> thisisanumber;
cin.ignore();
will read the input into thisisanumber and then discard the enter key. So the enter key counts as input as well? And so cin>> will only read the number and leave the rest and cin.ignore() will then read the enter key and discard it?
Also it says that
cin.get();
will just make the program wait for the user to press enter before continuing. This is much like read() from pascal but is this really the only thing cin.get does?
Oh and what do I use to divide 2 integers and get a float? Because this:
int x,y;
float k1,k2;
k1=x/y;
k2=y/x;
cout<<k1<<"\n"<<k2;
gives me integers as k1 and k2.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 14 at 09:09 UTC
— Ed. 2009 Aug 14 at 13:43 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
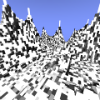

2007 Oct 19 • 5486
57,583 ₧
|
Because x and y are both integers the division performed will be integer as well, so 5/3 is 1. This is then implicitly cast to a float because you're assigning it to k1, I think. As in k1 = 1.0, which is obviously slightly different.
I think if you try using a.f and b.f it might work. No, it won't, that's C. C++ thinks I mean class members when I do that. SJ might know a way around this. Let's see:
#include <iostream>
int main()
{
int a=5,b=3;
float ratio=a/b;
std::cout <<ratio<<std::endl;
return 0;
}
prints out 1.
You'll have to do something like:
float temp1 = a,temp2 = b;ratio = temp1/temp2;
which again through the magic of implicit casting will make life better.
A quick question: how much object-oriented programming have you done? If you understand what a class is it would help quite a lot with understanding the basics of C++'s IO libraries. Coming from Pascal, C might be the better option :)
My third and final edit recommends this as talking some sense. I might take a look at your site though. The one I've posted is... sensible. It doesn't overwhelm and the coding style greatly matches mine, which is happy-making.
|
|
|
|
≡
|
2009 Aug 15 at 01:02 UTC
— Ed. 2009 Aug 15 at 01:16 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
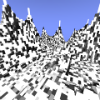

2007 Oct 19 • 5486
57,583 ₧
|
Since I said I'd not edit any more I'd recommend staying away from cin.ignore(). I can see that it is useful in some situations but using the redirect operator ( >> ) with cin does not require ignore().
|
|
|
|
≡
|
2009 Aug 15 at 01:20 UTC
|
|
|
|
Down Rodeo said: float temp1 = a,temp2 = b;ratio = temp1/temp2;
So I first 'transform' the integers into floats and then divide them? OK.
Down Rodeo said: A quick question: how much object-oriented programming have you done?
Well, actually I haven't really done any. I mean, I know what it is and understand its basic principals but I haven't really done any programming other than the simple procedural (or whatever it's called in english).
Down Rodeo said: If you understand what a class is it would help quite a lot with understanding the basics of C++'s IO libraries.
Hmmm... again I only know as much as my dad mentioned while explaining object-oriented programming to me.
Down Rodeo said: Coming from Pascal, C might be the better option :)
Yeah, I think I'm going to go for C first.
Down Rodeo said: Since I said I'd not edit any more I'd recommend staying away from cin.ignore(). I can see that it is useful in some situations but using the redirect operator ( >> ) with cin does not require ignore().
So what about cin.get()? And is "cin.get()" the same as "cin.get"?
P.S. Happy birthday.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 15 at 11:27 UTC
— Ed. 2009 Aug 15 at 15:03 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
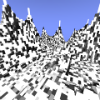

2007 Oct 19 • 5486
57,583 ₧
|
"cin.get()" is a member function of the class "cin". "cin.get", if it exists, would be some kind of variable, so I assume that I have missed out the brackets in one of my references to cin.get().
No, I didn't say it, so you saw it somewhere else. Um. If you were to use C instead of C++ then you could use the function "scanf()" to take input from stdin, which is usually the command line.
I feel I should ask: are you coding on Linux? If so, good on you. Makes life so much easier.
A last piece of info on istream::get(). I don't think you need to use it, not yet anyway.
If you do do C, we get to discuss pointers! Yay! They're awesome, you can do clever things with them. For instance ask me about how I used pointers in an obscure and pointless way when I could have used modulus operations!
And thanks :)
|
|
|
|
≡
|
2009 Aug 16 at 00:36 UTC
— Ed. 2009 Aug 16 at 01:02 UTC
|
|
|
|
Down Rodeo said: "cin.get()" is a member function of the class "cin". "cin.get", if it exists, would be some kind of variable, so I assume that I have missed out the brackets in one of my references to cin.get().
No, I didn't say it, so you saw it somewhere else. Um. If you were to use C instead of C++ then you could use the function "scanf()" to take input from stdin, which is usually the command line.
No, I was just curious because in pascal read = read() and write = write() etc.
Down Rodeo said: I feel I should ask: are you coding on Linux? If so, good on you. Makes life so much easier.
Well I wasn't until now, but I could move to linux now that I'm going to start with C.
Down Rodeo said: If you do do C, we get to discuss pointers! Yay! They're awesome, you can do clever things with them. For instance ask me about how I used pointers in an obscure and pointless way when I could have used modulus operations!
Did you ever use pointers in an obscure and pointless way when you could have used modulus operations? If so, please elaborate.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 16 at 13:53 UTC
— Ed. 2009 Aug 16 at 13:53 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
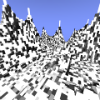

2007 Oct 19 • 5486
57,583 ₧
|
So basically, there was a programming task to find the last ten digits of some number, quite a large one, and the best way to do this was with the mod operation, i.e. the remainder when dividing one number by another (5 % 3 = 2). But if you didn't want to use the mod operation you could in fact do it like this:
Random code!
If you understand this, you'll have about the same understanding of C as me :D
Also that website is really good for documentation on functions. It's clear and concise and if you don't understand what it is saying you can look up other things to do with the function and if you don't understand those then you shouldn't be using the function :D
Sorry, it's huge, I'll delete it once you've read it. The code tags are fairly necessary.
|
|
|
|
≡
|
2009 Aug 16 at 14:14 UTC
— Ed. 2009 Aug 17 at 01:39 UTC
|
|
|
|
So basically what you did was you took only the last ten digits at all times and use only them for calculations. But I don't really understand what this line does:
prime = ( prime * 28433LL) + (unsigned long long int)1; /* Once it has been taken to the appropriate power, multiply it and add. */
What's 28433LL and what is (unsigned long long int)1.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 16 at 14:42 UTC
— Ed. 2009 Aug 16 at 14:56 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
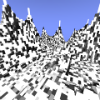

2007 Oct 19 • 5486
57,583 ₧
|
The appended LL means that the number preceding it should be a long long integer. They are unsigned by default I think. The bit preceding the 1 means "cast the integer 1 to a long long unsigned integer".
|
|
|
|
≡
|
2009 Aug 16 at 14:47 UTC
|
|
|
|
Down Rodeo said: The appended LL means that the number preceding it should be a long long integer. They are unsigned by default I think. The bit preceding the 1 means "cast the integer 1 to a long long unsigned integer".
Shouldn't it be just "unsigned long long int 1" then? Like "int a" or "float adsf"
And why do you need to multiply the prime by anything, isn't the prime supposed to be 2^something - 1? Meaning that you should only do prime = prime - 1;?
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 16 at 14:50 UTC
— Ed. 2009 Aug 16 at 14:57 UTC
|
|
|
|
*head explodes*
I drink to forget but I always remember.
|
|
|
|
≡
|
2009 Aug 16 at 16:59 UTC
|
|
|
|
#include <iscloudretarded>
int main()
{
int info,clouds_brain;
sumthing clouds_head;
cin>>info;
if (info>clouds_brain)
{
headsplosion(clouds_head);
cout<<"Headsplosion successful.";
}
cin.get();
return 0;
}
Anyway is there a certain compiler that I ought to be using for C (on Ubuntu) or do I just get myself a random one from google?
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 16 at 19:48 UTC
— Ed. 2009 Aug 16 at 19:54 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
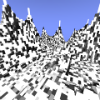

2007 Oct 19 • 5486
57,583 ₧
|
Mate de Vita said: #include "iscloudretarded.h"
int main()
{
int info,clouds_brain;
sumthing clouds_head;
cin>>info;
if (info>clouds_brain)
{
headsplosion(clouds_head);
cout<<"Headsplosion successful.";
}
cin.get();
return 0;
}
See I just don't understand why that's there. Whatever.
Mate de Vita said: Anyway is there a certain compiler that I ought to be using for C (on Ubuntu) or do I just get myself a random one from google?
I'd recommend GCC, the GNU Compiler Collection. Or the GNU C Compiler. It varies :D
Open a friendly console, type "sudo apt-get build-essential" and that will get you pretty much all you need. Unless I'm wrong.
Ah, your earlier questions... By default, 1 has a type int. But we want it to be a long long unsigned int, so we need to "cast" it as such, by sticking (long long unsigned int) in front. The thing you suggest sounds like you are confusing it with assignment. That is like saying "long long unsigned int fishes_in_the_sea = 1; which we can then cast to lots of different things. Like long long signed int if we want negative numbers and so on.
This explains the primes thing.
|
|
|
|
≡
|
2009 Aug 17 at 01:46 UTC
— Ed. 2009 Aug 17 at 01:47 UTC
|
|
|
|
Down Rodeo said: See I just don't understand why that's there. Whatever.
Just so you can see the "Headsplosion successful" before the program terminates.
Down Rodeo said: "I'd recommend GCC, the GNU Compiler Collection. Or the GNU C Compiler. It varies :D
Open a friendly console, type "sudo apt-get build-essential" and that will get you pretty much all you need. Unless I'm wrong."
It says E: Invalid operation build-essential
Down Rodeo said: Ah, your earlier questions... By default, 1 has a type int. But we want it to be a long long unsigned int, so we need to "cast" it as such, by sticking (long long unsigned int) in front. The thing you suggest sounds like you are confusing it with assignment. That is like saying "long long unsigned int fishes_in_the_sea = 1; which we can then cast to lots of different things. Like long long signed int if we want negative numbers and so on.
Ah ok, yes, I was confusing the two. All clear now.
Down Rodeo said: This explains the primes thing.
Ah, I see.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 17 at 06:41 UTC
— Ed. 2009 Aug 17 at 15:51 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
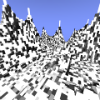

2007 Oct 19 • 5486
57,583 ₧
|
sudo apt-get install build-essential
My bad, I forgot you need to tell apt to install the package. I think I get what you mean by the cin.get() thing. You don't need this on Linux though as you are doing everything from the terminal anyway so all messages are saved in a scrollable history. You know what I mean, you're buggering around with apt anyway :D
|
|
|
|
≡
|
2009 Aug 17 at 11:47 UTC
|
|
|
|
Just as a note, before moving to C I had to finish my first ever program in C++:
#include <iostream>
using namespace std;
int main()
{
int x,y,v,r1,r2,p; //x,y=stevila, v=vsota, r=razlika, p=produkt
float k1,k2; //k=kolicnik
int po1,po2; //po=potenca
char crka;
do{
cout <<"Please insert a number:\n";
cin >>x;
cout <<"Please insert a second number:\n";
cin >>y;
v=x+y;
r1=x-y;
r2=y-x;
p=x*y;
float temp1 = x,temp2 = y;k1 = temp1/temp2; k2 = temp2/temp1;
po1=x;
po2=y;
for (int z=0; z<y-1; z++) {po1=po1*x;}
for (int z=0; z<x-1; z++) {po2=po2*y;}
cout <<x<<"+"<<y<<"="<<v<<"\n"<<x<<"-"<<y<<"="<<r1<<"\n"<<y<<"-"<<x<<"="<<r2<<"\n"<<x<<"*"<<y<<"="<<p<<"\n"<<x<<"/"<<y<<"="<<k1;
cout <<"\n"<<y<<"/"<<x<<"="<<k2<<"\n"<<x<<"^"<<y<<"="<<po1<<"\n"<<y<<"^"<<x<<"="<<po2<<"\n";
cin.get();
cout <<"If you would like the program to start again, write Y and press ENTER.\n";
cin >>crka;
}while (crka=='y'||crka=='Y');}
And it works.
Now on to start with C.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 17 at 15:57 UTC
— Ed. 2009 Aug 17 at 15:59 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
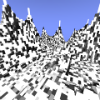

2007 Oct 19 • 5486
57,583 ₧
|
Fair enough. It seems sound, but if ever you want to make a program that is not for your own use, be careful with user input. People have been known to put some crazy shit in there...
Also, remember those Project Euler links I gave you? I'd recommend them as a great way to get started in the language. They can teach you recursion and useful tricks for speeding programs up. I guess you could post here or in the truck I made.
|
|
|
|
≡
|
2009 Aug 18 at 01:18 UTC
|
|
|
|
Down Rodeo said: Fair enough. It seems sound, but if ever you want to make a program that is not for your own use, be careful with user input. People have been known to put some crazy shit in there...
What do you mean by that?
Down Rodeo said: Also, remember those Project Euler links I gave you? I'd recommend them as a great way to get started in the language. They can teach you recursion and useful tricks for speeding programs up. I guess you could post here or in the truck I made.
Yeah, I'll do the problems there, but first I need to learn the basic syntax of C :) Mostly for arrays because I'll be needing them in the first problem, I think. If I'm to do it like my head is telling me to do it.
Also is there a certain text editor that I can use that's made for the purpose of programming? Because I'm used to having a text editor that e.g. automatically makes 2 spaces or a tab or whatever you're using when you go to a new line and stuff like that.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 18 at 09:02 UTC
— Ed. 2009 Aug 18 at 13:14 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
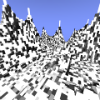

2007 Oct 19 • 5486
57,583 ₧
|
Mate de Vita said: What do you mean by that?
If you're looking for an int and someone puts in, say, a number that is too large or "fbeifb\s" then that will cause problems.
Mate de Vita said: Yeah, I'll do the problems there, but first I need to learn the basic syntax of C :) Mostly for arrays because I'll be needing them in the first problem, I think. If I'm to do it like my head is telling me to do it.
You could do it like that. You don't have to - in fact it is entirely possible to do it with loops and control variables. But I'd be interested to see your solution. For what it's worth arrays are defined like int myIntArray[50] Remember that the elements are numbered from 0.
Mate de Vita said: Also is there a certain text editor that I can use that's made for the purpose of programming? Because I'm used to having a text editor that e.g. automatically makes 2 spaces or a tab or whatever you're using when you go to a new line and stuff like that.
Eh, there are loads. I use Gedit mainly but it isn't the most comprehensive of editors. There's also Code::Blocks, Vim and various distribution-specific editors.
|
|
|
|
≡
|
2009 Aug 18 at 17:31 UTC
|
|
|
|
Down Rodeo said: You could do it like that. You don't have to - in fact it is entirely possible to do it with loops and control variables. But I'd be interested to see your solution. For what it's worth arrays are defined like int myIntArray[50] Remember that the elements are numbered from 0.
I was thinking something like a for loop that checks every number from 1 to 1000 if it gives anything when modded (spelling lol?) by (with?) 3 and 5. If either of those mods is zero it writes the number into the array[z] and then does z++ (so that the next number is written into the next field).
Then another for loop, this time for z and just do number+=array[z] (or whatever the syntax for that is).
Another way I thought of is first a while loop that does while y<1000 y+=3 z++ array[z]=y and then another while loop that does the same for 5, only that it checks for every number if it's been written into the array before. Then it finishes the same way as the previous one.
And yes, I do usually complicate where there's no need to. That's one of the main flaws I need to get rid of if I'm to be any good at programming.
Also I may have made a few mistakes right now, I'm a bit tired after a 2-hour training and a 1 and a half hour walk home afterwards while pushing a bike with a flat tire.
I'll get to this tomorrow, right now I think I'm going to just fall down asleep.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 18 at 21:28 UTC
— Ed. 2009 Aug 18 at 21:34 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
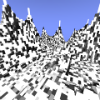

2007 Oct 19 • 5486
57,583 ₧
|
There is little better in this life than a good sleep. Yes, those are the two main ways of doing it. You are spot on with both. Obviously the latter solution is better as it involves fewer if-checks and modulus operations. It only has about 500 ifs, compared to 6000 for the for loop version. I think, I'm just guessing here. I assume you know that all for loops can be written as whiles?
Wait, no, you are being silly with them both. Why store the numbers in the array? Why not just add them, seeing as the sum is all you're looking for? That way you avoid the check. Tell you what, if you want to post your solution I can show you mine afterwards which is about as small as I can make it.
Wait, no, the reason you are checking to see if it has been in before is for the numbers that are multiples of both three and five. As you were.
|
|
|
|
≡
|
2009 Aug 19 at 14:12 UTC
— Ed. 2009 Aug 19 at 14:14 UTC
|
|
|
|
I installed Code::Blocks now but I can't run it... How do I run it?
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 19 at 17:04 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
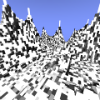

2007 Oct 19 • 5486
57,583 ₧
|
Applications -> programming. I assume you used the Add/remove programs tool?
|
|
|
|
≡
|
2009 Aug 19 at 17:09 UTC
|
|
|
|
Down Rodeo said: Applications -> programming. I assume you used the Add/remove programs tool?
Nope, terminal commands, I copied them off of their wiki. Anyway it is right where you said it'd be. Amazing.
...and that's the bottom line because Mate de Vita said so.
|
|
|
|
≡
|
2009 Aug 19 at 17:12 UTC
— Ed. 2009 Aug 19 at 17:12 UTC
|
|
|
Down Rodeo
Cap'n Moth of the Firehouse
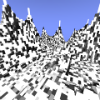

2007 Oct 19 • 5486
57,583 ₧
|
It's a very well put together OS. If I could remember the kind of things I'd set up on my machine I'd let you know but as it is I have forgotten the customisations I've made.
For some reason I just triple posted. I didn't think that was possible. Ah well.
|
|
|
|
≡
|
2009 Aug 19 at 18:15 UTC
|
|
|
|
|